Java用自带的Image IO给图片添加水印
import sun.font.FontDesignMetrics;import javax.imageio.ImageIO;import java.awt.*;import java.awt.image.BufferedImage;import java.io.File;import java.io.FileOutputStream;import java.io.IOException;/** * @Author ChengJianSheng * @Date 2021/6/10 */public class WatermarkUtil{ public static void main(String[] args) throws IOException{ addText('F:/1.jpeg', '我的梦想是成为火影');}/** * 加文字水印 * @param srcPath 原文件路径 * @param content 文字内容 * @throws IOException */ public static void addText(String srcPath, String content) throws IOException {// 读取原图片信息BufferedImage srcImage = ImageIO.read(new File(srcPath));int width = srcImage.getWidth();int height = srcImage.getHeight();// 创建画笔,设置绘图区域BufferedImage bufferedImage = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);Graphics2D g = bufferedImage.createGraphics();g.drawImage(srcImage, 0, 0, width, height, null);//g.drawImage(srcImage.getScaledInstance(width, height, Image.SCALE_SMOOTH), 0, 0, null);g.setFont(new Font('宋体', Font.PLAIN, 32));g.setColor(Color.RED);// 计算文字长度int len = g.getFontMetrics(g.getFont()).charsWidth(content.toCharArray(), 0, content.length());FontDesignMetrics metrics = FontDesignMetrics.getMetrics(g.getFont());int len2 = metrics.stringWidth(content);System.out.println(len);System.out.println(len2);// 计算文字坐标int x = width - len - 10;int y = height - 20;//int x = width - 2 * len;//int y = height - 1 * len;g.drawString(content, x, y);g.dispose();// 输出文件FileOutputStream fos = new FileOutputStream('F:/2.png');ImageIO.write(bufferedImage, 'png', fos);fos.flush();fos.close(); }}
可以设置文字透明度
g.setComposite(AlphaComposite.getInstance(AlphaComposite.SRC_ATOP, 0.8f)); 2. 旋转文字
import sun.font.FontDesignMetrics;import javax.imageio.ImageIO;import java.awt.*;import java.awt.geom.AffineTransform;import java.awt.image.BufferedImage;import java.io.File;import java.io.FileOutputStream;import java.io.IOException;/** * @Author ChengJianSheng * @Date 2021/6/10 */public class WatermarkUtil{ // 水印透明度 private static final float alpha = 0.5 f; // 水印文字字体 private static final Font font = new Font('宋体', Font.BOLD, 30); // 水印文字颜色 private static final Color color = Color.RED; public static void main(String[] args) throws IOException{ addText('F:/1.jpeg', '图片由木叶村提供,仅供忍者联军使用!');}/** * 加文字水印 * @param srcPath 原文件路径 * @param content 文字内容 * @throws IOException */ public static void addText(String srcPath, String content) throws IOException {// 读取原图片信息BufferedImage srcImage = ImageIO.read(new File(srcPath));int width = srcImage.getWidth();int height = srcImage.getHeight();// 创建画笔,设置绘图区域BufferedImage bufferedImage = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);Graphics2D g = bufferedImage.createGraphics();g.drawImage(srcImage, 0, 0, width, height, null);//g.drawImage(srcImage.getScaledInstance(width, height, Image.SCALE_SMOOTH), 0, 0, null);// 旋转文字AffineTransform affineTransform = g.getTransform();affineTransform.rotate(Math.toRadians(-30), 0, 0);Font rotatedFont = font.deriveFont(affineTransform);g.setFont(rotatedFont); // 字体g.setColor(color); // 颜色g.setComposite(AlphaComposite.getInstance(AlphaComposite.SRC_ATOP, alpha)); // 透明度// 计算文字长度int len = g.getFontMetrics(g.getFont()).charsWidth(content.toCharArray(), 0, content.length());FontDesignMetrics metrics = FontDesignMetrics.getMetrics(g.getFont());int len2 = metrics.stringWidth(content);System.out.println(len);System.out.println(len2);// 计算水印文字坐标int x = width / 5;int y = height / 3 * 2;g.drawString(content, x, y);g.dispose();// 输出文件FileOutputStream fos = new FileOutputStream('F:/2.png');ImageIO.write(bufferedImage, 'png', fos);fos.flush();fos.close(); }}
画矩形框
import sun.font.FontDesignMetrics;import javax.imageio.ImageIO;import java.awt.*;import java.awt.image.BufferedImage;import java.io.File;import java.io.FileOutputStream;import java.io.IOException;/** * @Author ChengJianSheng * @Date 2021/6/10 */public class WatermarkUtil{ // 水印透明度 private static final float alpha = 0.5 f; // 水印文字字体 private static final Font font = new Font('宋体', Font.BOLD, 30); // 水印文字颜色 private static final Color color = Color.RED; public static void main(String[] args) throws IOException{ addText('F:/1.jpeg', '图片由木叶村提供,仅供忍者联军使用!');}/** * 加文字水印 * @param srcPath 原文件路径 * @param content 文字内容 * @throws IOException */ public static void addText(String srcPath, String content) throws IOException {// 读取原图片信息BufferedImage srcImage = ImageIO.read(new File(srcPath));int width = srcImage.getWidth();int height = srcImage.getHeight();// 创建画笔,设置绘图区域BufferedImage bufferedImage = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);Graphics2D g = bufferedImage.createGraphics();g.drawImage(srcImage, 0, 0, width, height, null);g.setFont(font); // 字体g.setColor(color); // 颜色g.setComposite(AlphaComposite.getInstance(AlphaComposite.SRC_ATOP, alpha)); // 透明度// 计算文字宽高度FontDesignMetrics metrics = FontDesignMetrics.getMetrics(font);int textWidth = metrics.stringWidth(content); // 文字宽度int textHeight = metrics.getHeight(); // 文字高度// 计算文字坐标int x = (width - textWidth) / 2;int y = (height + textHeight) / 2;// 写文字g.drawString(content, x, y);// 画矩形int padding = 10; // 内边距g.drawRect(x - padding / 2, y - textHeight, textWidth + padding, textHeight + padding);g.dispose();// 输出文件FileOutputStream fos = new FileOutputStream('F:/2.png');ImageIO.write(bufferedImage, 'png', fos);fos.flush();fos.close(); }}
import sun.font.FontDesignMetrics;import javax.imageio.ImageIO;import java.awt.*;import java.awt.image.BufferedImage;import java.io.File;import java.io.FileOutputStream;import java.io.IOException;/** * @Author ChengJianSheng * @Date 2021/6/10 */public class WatermarkUtil{ // 水印透明度 private static final float alpha = 0.5 f; // 水印文字字体 private static final Font font = new Font('宋体', Font.BOLD, 30); // 水印文字颜色 private static final Color color = Color.RED; public static void main(String[] args) throws IOException{ addText('F:/1.jpeg', '图片由木叶村提供,仅供忍者联军使用!');}/** * 加文字水印 * @param srcPath 原文件路径 * @param content 文字内容 * @throws IOException */ public static void addText(String srcPath, String content) throws IOException {// 读取原图片信息BufferedImage srcImage = ImageIO.read(new File(srcPath));int width = srcImage.getWidth();int height = srcImage.getHeight();// 创建画笔,设置绘图区域BufferedImage bufferedImage = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);Graphics2D g = bufferedImage.createGraphics();g.drawImage(srcImage, 0, 0, width, height, null);g.setFont(font); // 字体g.setColor(color); // 颜色g.setComposite(AlphaComposite.getInstance(AlphaComposite.SRC_ATOP, alpha)); // 透明度// 计算文字宽高度FontDesignMetrics metrics = FontDesignMetrics.getMetrics(font);int textWidth = metrics.stringWidth(content); // 文字宽度int textHeight = metrics.getHeight(); // 文字高度// 旋转坐标轴g.translate(-width / 5, height / 4);g.rotate(-30 * Math.PI / 180);// 计算文字坐标int x = (width - textWidth) / 2;int y = (height + textHeight) / 2;// 写文字g.drawString(content, x, y);// 画矩形int padding = 10; // 内边距g.drawRect(x - padding / 2, y - textHeight, textWidth + padding, textHeight + padding);g.dispose();// 输出文件FileOutputStream fos = new FileOutputStream('F:/2.png');ImageIO.write(bufferedImage, 'png', fos);fos.flush();fos.close(); }}
结合下载功能,完整的代码如下:
/** * 下载 */@GetMapping('/download')public void download(@RequestParam('mediaId') String mediaId, HttpServletRequest request, HttpServletResponse response) throws IOException{ SysFile sysFile = sysFileService.getByMediaId(mediaId); if(null == sysFile) {throw new IllegalArgumentException('文件不存在'); } String mimeType = request.getServletContext().getMimeType(sysFile.getPath()); System.out.println(mimeType); response.setContentType(sysFile.getContentType()); response.setHeader(HttpHeaders.CONTENT_DISPOSITION, 'attachment;filename=' + URLEncoder.encode(sysFile.getOriginalFilename(), 'UTF-8')); //FileInputStream fis = new FileInputStream(sysFile.getPath()); ServletOutputStream sos = response.getOutputStream(); WatermarkUtil.addText(sysFile.getPath(), '哈哈哈哈', sos); //IOUtils.copy(fis, sos); //IOUtils.closeQuietly(fis); IOUtils.closeQuietly(sos);}
import sun.font.FontDesignMetrics;import javax.imageio.ImageIO;import java.awt.*;import java.awt.image.BufferedImage;import java.io.File;import java.io.IOException;import java.io.OutputStream;/** * @Author ChengJianSheng * @Date 2021/6/10 */public class WatermarkUtil{ // 水印透明度 private static final float alpha = 0.5 f; // 水印文字字体 private static final Font font = new Font('宋体', Font.BOLD, 30); // 水印文字颜色 private static final Color color = Color.RED; /** * 加文字水印 * @param srcPath 原文件路径 * @param content 文字内容 * @throws IOException */ public static void addText(String srcPath, String content, OutputStream outputStream) throws IOException {// 读取原图片信息BufferedImage srcImage = ImageIO.read(new File(srcPath));int width = srcImage.getWidth();int height = srcImage.getHeight();// 画板BufferedImage bufferedImage = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);// 画笔Graphics2D g = bufferedImage.createGraphics();g.drawImage(srcImage, 0, 0, width, height, null);g.setFont(font); // 字体g.setColor(color); // 颜色g.setComposite(AlphaComposite.getInstance(AlphaComposite.SRC_ATOP, alpha)); // 透明度// 计算文字宽高度FontDesignMetrics metrics = FontDesignMetrics.getMetrics(font);int textWidth = metrics.stringWidth(content); // 文字宽度int textHeight = metrics.getHeight(); // 文字高度// 旋转坐标轴g.translate(-width / 5, height / 4);g.rotate(-30 * Math.PI / 180);// 计算文字坐标int x = (width - textWidth) / 2;int y = (height + textHeight) / 2;// 写文字g.drawString(content, x, y);// 画矩形int padding = 10; // 内边距g.drawRect(x - padding / 2, y - textHeight, textWidth + padding, textHeight + padding);g.dispose();// 输出ImageIO.write(bufferedImage, 'png', outputStream); }}另外的写法
import javax.imageio.ImageIO;import java.awt.*;import java.awt.image.BufferedImage;import java.io.File;import java.io.FileOutputStream;/** * @ProjectName: test * @Package: com.test.utils * @ClassName: MyTest * @Author: luqiming * @Description: * @Date: 2020/10/29 11:48 * @Version: 1.0 */public class AddWatermarkUtil { public static void waterPress(String srcImgPath, String outImgPath, Color markContentColor, int fontSize, String waterMarkContent) {try { String[] waterMarkContents = waterMarkContent.split('||'); // 读取原图片信息 File srcImgFile = new File(srcImgPath); Image srcImg = ImageIO.read(srcImgFile); int srcImgWidth = srcImg.getWidth(null); int srcImgHeight = srcImg.getHeight(null); // 加水印 BufferedImage bufImg = new BufferedImage(srcImgWidth, srcImgHeight, BufferedImage.TYPE_INT_RGB); // 得到画笔对象 Graphics2D g = bufImg.createGraphics(); // 设置起点 g.drawImage(srcImg, 0, 0, srcImgWidth, srcImgHeight, null); Font font = new Font('宋体', Font.PLAIN, fontSize); // 根据图片的背景设置水印颜色 g.setColor(markContentColor); // 设置水印文字字体 g.setFont(font); // 数组长度 int contentLength = waterMarkContents.length; // 获取水印文字中最长的 int maxLength = 0; for (int i = 0; i < contentLength; i++) {int fontlen = getWatermarkLength(waterMarkContents[i], g);if (maxLength < fontlen) { maxLength = fontlen;} } for (int j = 0; j < contentLength; j++) {waterMarkContent = waterMarkContents[j];int tempX = 10;int tempY = fontSize;// 单字符长度int tempCharLen = 0;// 单行字符总长度临时计算int tempLineLen = 0;StringBuffer sb = new StringBuffer();for (int i = 0; i < waterMarkContent.length(); i++) { char tempChar = waterMarkContent.charAt(i); tempCharLen = getCharLen(tempChar, g); tempLineLen += tempCharLen; if (tempLineLen >= srcImgWidth) {// 长度已经满一行,进行文字叠加g.drawString(sb.toString(), tempX, tempY);// 清空内容,重新追加sb.delete(0, sb.length());tempLineLen = 0; } // 追加字符 sb.append(tempChar);}// 通过设置后两个输入参数给水印定位g.drawString(sb.toString(), 20, srcImgHeight - (contentLength - j - 1) * tempY-50); } g.dispose(); // 输出图片 FileOutputStream outImgStream = new FileOutputStream(outImgPath); ImageIO.write(bufImg, 'jpg', outImgStream); outImgStream.flush(); outImgStream.close();} catch (Exception e) { e.printStackTrace();} } public static int getCharLen(char c, Graphics2D g) {return g.getFontMetrics(g.getFont()).charWidth(c); } /** * 获取水印文字总长度 * * @paramwaterMarkContent水印的文字 * @paramg * @return水印文字总长度 */ public static int getWatermarkLength(String waterMarkContent, Graphics2D g) {return g.getFontMetrics(g.getFont()).charsWidth(waterMarkContent.toCharArray(), 0, waterMarkContent.length()); } public static void main(String[] args) {// 原图位置, 输出图片位置, 水印文字颜色, 水印文字String font = '张天爱||就很完美||2020-05-27 17:00:00';String inputAddress = 'F:/UpupooWallpaper/1.jpg';String outputAddress = 'F:/UpupooWallpaper/1.jpg';Color color = Color.GREEN;waterPress(inputAddress, outputAddress, color, 50, font); }}
添加效果
关于水印位置,需要修改:
//左下角 g.drawString(sb.toString(), 0, srcImgHeight - (contentLength - j - 1) * tempY);//右下角 g.drawString(sb.toString(), srcImgWidth - maxLength, srcImgHeight - (contentLength - j - 1) * tempY);
以上就是Java用自带的Image IO给图片添加水印的详细内容,更多关于Java 图片添加水印的资料请关注好吧啦网其它相关文章!
相关文章:
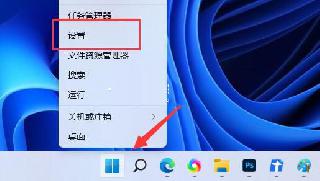