java HttpClient传输json格式的参数实例讲解
最近的一个接口项目,传的参数要求是json,需要特殊处理一下。
重点是这两句话:httpPost.setHeader('Content-Type', 'application/json;charset=UTF-8');se.setContentType(CONTENT_TYPE_TEXT_JSON);
这两句话的作用与jmeter的设置header信息类似
package com.base;import java.io.UnsupportedEncodingException;import org.apache.http.HttpEntity;import org.apache.http.client.methods.CloseableHttpResponse;import org.apache.http.client.methods.HttpPost;import org.apache.http.entity.StringEntity;import org.apache.http.impl.client.DefaultHttpClient;import org.apache.http.impl.conn.PoolingClientConnectionManager;import org.apache.http.util.EntityUtils;/** * @author QiaoJiafei * @version 创建时间:2015年11月4日 下午1:55:45 * 类说明 */public class HttpGetByJson { public static void main(String args[]) throws Exception{ final String CONTENT_TYPE_TEXT_JSON = 'text/json'; DefaultHttpClient client = new DefaultHttpClient( new PoolingClientConnectionManager()); String url = 'http://172.16.30.226:8091/svc/authentication/register'; String js = '{'userName':'18600363833','validateChar':'706923','randomChar':'706923','password':'123456','confirmPwd':'123456','recommendMobile':'','idCard':'320601197608285792','realName':'阙岩','verifyCode'}'; HttpPost httpPost = new HttpPost(url); httpPost.setHeader('Content-Type', 'application/json;charset=UTF-8'); StringEntity se = new StringEntity(js); se.setContentType(CONTENT_TYPE_TEXT_JSON); httpPost.setEntity(se); CloseableHttpResponse response2 = null; response2 = client.execute(httpPost); HttpEntity entity2 = null; entity2 = response2.getEntity(); String s2 = EntityUtils.toString(entity2, 'UTF-8'); System.out.println(s2); } }
补充:HttpClient以json形式的参数调用http接口并对返回的json数据进行处理(可以带文件)
1、参数的url就是被调用的地址,map是你要传的参数。参数转成json我使用的是gson方式转换的。
主要使用的jar包有httpclient-4.5.3.jar、httpcore-4.4.6.jar、commons-codec-1.9.jar、gson-2.2.4.jar和commons-logging-1.2.jar。
如果发送的post请求想传送文件,需添加httpmime-4.5.3.jar包,并设置如下代码:
HttpEntity multipartEntityBuilder = MultipartEntityBuilder.create().addBinaryBody('file', new File('D:workspaceprogrammWebContentprogramm1991.zip')).build();
第一个参数表示请求字段名,第二个参数就是文件。
还想添加参数则
HttpEntity multipartEntityBuilder = MultipartEntityBuilder.create().addTextBody('name', '张三').addBinaryBody('file', new File('D:workspaceprogrammWebContentprogramm1991.zip')).build();httpPost.setEntity(multipartEntityBuilder);
import java.io.IOException;import java.util.Map;import org.apache.http.HttpEntity;import org.apache.http.client.ClientProtocolException;import org.apache.http.client.methods.CloseableHttpResponse;import org.apache.http.client.methods.HttpPost;import org.apache.http.entity.StringEntity;import org.apache.http.impl.client.CloseableHttpClient;import org.apache.http.impl.client.HttpClients;import org.apache.http.util.EntityUtils;import com.google.gson.Gson;public class HttpClientUtil { private final static String CONTENT_TYPE_TEXT_JSON = 'text/json'; public static String postRequest(String url, Map<String, Object> param) throws ClientProtocolException, IOException{ CloseableHttpClient client = HttpClients.createDefault(); HttpPost httpPost = new HttpPost(url); httpPost.setHeader('Content-Type', 'application/json;charset=UTF-8'); Gson gson = new Gson(); String parameter = gson.toJson(param); StringEntity se = new StringEntity(parameter); se.setContentType(CONTENT_TYPE_TEXT_JSON); httpPost.setEntity(se); CloseableHttpResponse response = client.execute(httpPost); HttpEntity entity = response.getEntity(); String result = EntityUtils.toString(entity, 'UTF-8'); return result; }}
2、返回的结果也可以使用gson转换成对象进行下一步操作。
import com.google.gson.Gson;public class GsonUtil { public static <T> T jsonToObject(String jsonData, Class<T> type) { Gson gson = new Gson(); T result = gson.fromJson(jsonData, type); return result; } public static void main(String[] args) { String json = '{’id’:’1’,’name’:’zhang’,’address’:’Hubei’}'; jsonToObject(json, Person.class); Person person = jsonToObject(json, Person.class); System.out.println(person); }}
建立要转成的对象的类。
import java.util.Date;public class Person { private int id; private String name; private int age; private String address;public int getId() { return id; } public void setId(int id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } public String getAddress() { return address; } public void setAddress(String address) { this.address = address; } @Override public String toString() { return 'Person [id=' + id + ', name=' + name + ', age=' + age + ', address=' + address + ']'; }}
3、发送以键值对形式的参数的post请求
package com.avatarmind.httpclient;import java.util.ArrayList;import java.util.List;import org.apache.http.HttpEntity;import org.apache.http.NameValuePair;import org.apache.http.client.entity.UrlEncodedFormEntity;import org.apache.http.client.methods.CloseableHttpResponse;import org.apache.http.client.methods.HttpPost;import org.apache.http.impl.client.CloseableHttpClient;import org.apache.http.impl.client.HttpClients;import org.apache.http.message.BasicNameValuePair;import org.apache.http.util.EntityUtils;public class HttpClient3 { public static void main(String[] args) throws Exception { CloseableHttpClient client = HttpClients.createDefault(); String url = 'http://yuntuapi.amap.com/datamanage/table/create'; HttpPost httpPost = new HttpPost(url); // 参数形式为key=value&key=value List<NameValuePair> formparams = new ArrayList<NameValuePair>(); formparams.add(new BasicNameValuePair('key', '060212638b94290e3dd0648c15753b64')); formparams.add(new BasicNameValuePair('name', '火狐')); // 加utf-8进行编码 UrlEncodedFormEntity uefEntity = new UrlEncodedFormEntity(formparams, 'UTF-8'); httpPost.setEntity(uefEntity); CloseableHttpResponse response = client.execute(httpPost); HttpEntity entity = response.getEntity(); String result = EntityUtils.toString(entity, 'UTF-8'); System.out.println(result); }}
以上为个人经验,希望能给大家一个参考,也希望大家多多支持好吧啦网。如有错误或未考虑完全的地方,望不吝赐教。
相关文章:
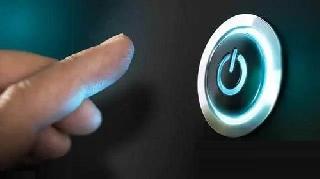