Java 网络编程之 TCP 实现简单的聊天系统
客户端
1、连接服务器 Socket
2、发送消息
package lesson02;import java.io.IOException;import java.io.OutputStream;import java.net.InetAddress;import java.net.Socket;/** * 客户端 */public class TcpClientDemo1 { public static void main(String[] args) { Socket socket = null; OutputStream os = null; try { //1、要知道服务器的地址 端口号 InetAddress serverIP = InetAddress.getByName('127.0.0.1'); int port = 9999; //2、创建一个 socket 连接 socket = new Socket(serverIP, port); //3、发送消息 IO流 os = socket.getOutputStream(); os.write('你好,欢迎学习狂神学Java'.getBytes()); } catch (Exception e) { e.printStackTrace(); } finally { if (os != null){try { os.close();} catch (IOException e) { e.printStackTrace();} } if (socket != null){try { socket.close();} catch (IOException e) { e.printStackTrace();} } } }}
服务端
1、建立服务的端口 ServerSocket
2、等待用户的连接 accept
3、接收用户的消息
package lesson02;import java.io.ByteArrayOutputStream;import java.io.IOException;import java.io.InputStream;import java.net.ServerSocket;import java.net.Socket;/** * 服务端 */public class TcpServerDemo1 { public static void main(String[] args) { ServerSocket serverSocket = null; Socket socket = null; InputStream is = null; ByteArrayOutputStream baos = null; try { //1、我得有一个地址 serverSocket = new ServerSocket(9999); while (true){//2、等待客户端连接过来socket = serverSocket.accept();//3、读取客户端的消息is = socket.getInputStream();//管道流baos = new ByteArrayOutputStream();byte[] buffer = new byte[1024];int len;while((len = is.read(buffer)) != -1){ baos.write(buffer, 0 , len);}System.out.println(baos.toString()); } /* byte[] buffer = new byte[1024]; int len; while ((len = is.read(buffer)) != -1){String msg = new String(buffer, 0, len);System.out.println(msg); } */ } catch (IOException e) { e.printStackTrace(); } finally { //关闭资源 if (baos != null){try { baos.close();} catch (IOException e) { e.printStackTrace();} } if (is != null){try { is.close();} catch (IOException e) { e.printStackTrace();} } if (socket != null){try { socket.close();} catch (IOException e) { e.printStackTrace();} } if (serverSocket != null){try { serverSocket.close();} catch (IOException e) { e.printStackTrace();} } } }}
服务端
1、建立服务的端口 ServerSocket
2、等待用户的连接 accept
3、接收用户的消息
package lesson02;import java.io.ByteArrayOutputStream;import java.io.IOException;import java.io.InputStream;import java.net.ServerSocket;import java.net.Socket;/** * 服务端 */public class TcpServerDemo1 { public static void main(String[] args) { ServerSocket serverSocket = null; Socket socket = null; InputStream is = null; ByteArrayOutputStream baos = null; try { //1、我得有一个地址 serverSocket = new ServerSocket(9999); while (true){//2、等待客户端连接过来socket = serverSocket.accept();//3、读取客户端的消息is = socket.getInputStream();//管道流baos = new ByteArrayOutputStream();byte[] buffer = new byte[1024];int len;while((len = is.read(buffer)) != -1){ baos.write(buffer, 0 , len);}System.out.println(baos.toString()); } /* byte[] buffer = new byte[1024]; int len; while ((len = is.read(buffer)) != -1){String msg = new String(buffer, 0, len);System.out.println(msg); } */ } catch (IOException e) { e.printStackTrace(); } finally { //关闭资源 if (baos != null){try { baos.close();} catch (IOException e) { e.printStackTrace();} } if (is != null){try { is.close();} catch (IOException e) { e.printStackTrace();} } if (socket != null){try { socket.close();} catch (IOException e) { e.printStackTrace();} } if (serverSocket != null){try { serverSocket.close();} catch (IOException e) { e.printStackTrace();} } } }}
以上就是Java 网络编程之 TCP 实现简单的聊天系统的详细内容,更多关于Java 实现简单的聊天系统的资料请关注好吧啦网其它相关文章!
相关文章:
1. IDEA 重新导入依赖maven 命令 reimport的方法2. Docker究竟是什么 为什么这么流行 它的优点和缺陷有哪些?3. idea打开多个窗口的操作方法4. Intellij IDEA 阅读源码的 4 个绝技(必看)5. JavaScript实现网页版五子棋游戏6. Java14发布了,再也不怕NullPointerException了7. 如何通过vscode运行调试javascript代码8. IntelliJ IDEA 统一设置编码为utf-8编码的实现9. IntelliJ IDEA 2020.2正式发布,两点多多总能助你提效10. IntelliJ IDEA设置编码格式的方法
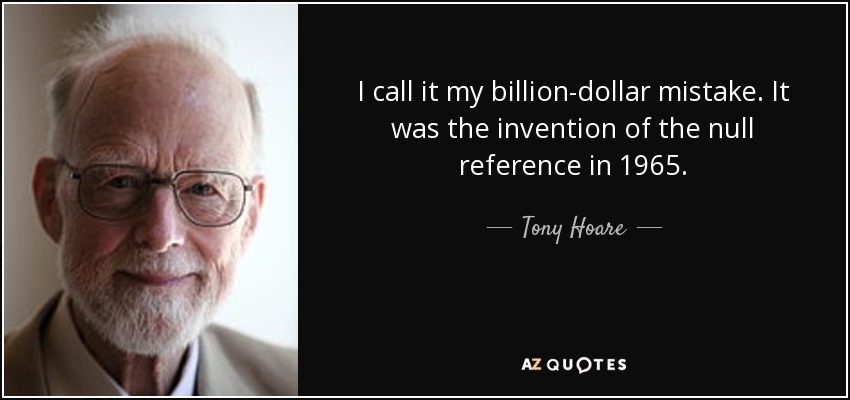