java 将字符串、list 写入到文件,并读取内容的案例
我就废话不多说了,大家还是直接看代码吧~
import java.io.BufferedReader;import java.io.BufferedWriter;import java.io.FileInputStream;import java.io.FileNotFoundException;import java.io.FileOutputStream;import java.io.FileWriter;import java.io.IOException;import java.io.InputStreamReader;import java.io.ObjectInputStream;import java.io.ObjectOutputStream;import java.io.OutputStreamWriter;import java.io.StreamCorruptedException;import java.io.UnsupportedEncodingException;import java.util.List; import android.graphics.Bitmap; public class FileUtils { /** * 字符流写入字符串到txt */@SuppressWarnings('resource')public static void FileString(String path, String data) {try {FileWriter writer = new FileWriter(path);// 字符流writer.write(data);writer.close();} catch (IOException e) {// TODO Auto-generated catch blocke.printStackTrace();}} /** * 字节输出到txt * * @param path * @param data */@SuppressWarnings('resource')public static void FileString2(String path, String data) {try {FileOutputStream outputStream = new FileOutputStream(path);// 字节流outputStream.write(data.getBytes());outputStream.close();} catch (FileNotFoundException e) {// TODO Auto-generated catch blocke.printStackTrace();} catch (IOException e) {// TODO Auto-generated catch blocke.printStackTrace();}} /** * 设置编码格式写出到txt * * @param path * @param data */public static void FileString3(String path, String data) {try {OutputStreamWriter writer = new OutputStreamWriter(new FileOutputStream(path), 'UTF-8');// 设置编码格式writer.write(data);writer.close();} catch (UnsupportedEncodingException e) {// TODO Auto-generated catch blocke.printStackTrace();} catch (IOException e) {// TODO Auto-generated catch blocke.printStackTrace();}} /** * 追加写入到txt * * @param path * @param data */@SuppressWarnings('resource')public static void FileString4(String path, String data) {try {FileOutputStream outputStream = new FileOutputStream(path, true);// 追加写入outputStream.write(('rn' + data).getBytes());outputStream.close();} catch (FileNotFoundException e) {// TODO Auto-generated catch blocke.printStackTrace();} catch (IOException e) {// TODO Auto-generated catch blocke.printStackTrace();}} /** * 存储list到文件 * * @param path * @param list */@SuppressWarnings('resource')public static <T> void FileWriteList1(String path, List<T> list) {try {FileOutputStream outputStream = new FileOutputStream(path);ObjectOutputStream stream = new ObjectOutputStream(outputStream);stream.writeObject(list);stream.close();outputStream.close();} catch (FileNotFoundException e) {// TODO Auto-generated catch blocke.printStackTrace();} catch (IOException e) {// TODO Auto-generated catch blocke.printStackTrace();}} /** * 设置编码格式存储list到txt * * @param path * @param list */ @SuppressWarnings('resource')public static <T> void FileWriteList(String path, List<T> list) {try {BufferedWriter bufferedWriter = new BufferedWriter(new OutputStreamWriter(new FileOutputStream(path), 'UTF-8'));for (T s : list) {bufferedWriter.write(s.toString());bufferedWriter.newLine();bufferedWriter.flush();}bufferedWriter.close();} catch (UnsupportedEncodingException e) {// TODO Auto-generated catch blocke.printStackTrace();} catch (FileNotFoundException e) {// TODO Auto-generated catch blocke.printStackTrace();} catch (IOException e) {// TODO Auto-generated catch blocke.printStackTrace();}} /** * bitmap 写入到本地 * * @param path * @param bitmap */@SuppressWarnings('resource')public static void FileBitmap(String path, Bitmap bitmap) {try {FileOutputStream outputStream = new FileOutputStream(path);bitmap.compress(Bitmap.CompressFormat.PNG, 100, outputStream);outputStream.flush();outputStream.close();} catch (FileNotFoundException e) {// TODO Auto-generated catch blocke.printStackTrace();} catch (IOException e) {// TODO Auto-generated catch blocke.printStackTrace();}} /** * 读取本地文件数据设置指定编码 * * @param path */@SuppressWarnings('resource')public static String FileInputString(String path) {StringBuffer buffer = new StringBuffer();try {BufferedReader reader = new BufferedReader(new InputStreamReader(new FileInputStream(path), 'UTF-8'));String data = null;while ((data = reader.readLine()) != null) {buffer.append(data + 'rn');}reader.close();} catch (UnsupportedEncodingException e) {// TODO Auto-generated catch blocke.printStackTrace();} catch (FileNotFoundException e) {// TODO Auto-generated catch blocke.printStackTrace();} catch (IOException e) {// TODO Auto-generated catch blocke.printStackTrace();}return buffer.toString();} /** * 根据字节读取文件 * * @param path * @return */@SuppressWarnings('resource')public static String FileInputString2(String path) {StringBuffer buffer = new StringBuffer();try {FileInputStream inputStream = new FileInputStream(path);byte[] bytes = new byte[1024];int bytead = 0;while ((bytead = inputStream.read(bytes)) != -1) {buffer.append(new String(bytes, 0, bytead));}inputStream.close(); } catch (FileNotFoundException e) {// TODO Auto-generated catch blocke.printStackTrace();} catch (IOException e) {// TODO Auto-generated catch blocke.printStackTrace();}return buffer.toString();} /** * 获取本地文件中的list * * @param path */ @SuppressWarnings('resource')public static <T> void FileInputList(String path) {try {FileInputStream inputStream = new FileInputStream(path);ObjectInputStream stream = new ObjectInputStream(inputStream);List<T> list = (List<T>) stream.readObject();inputStream.close();stream.close();} catch (FileNotFoundException e) {// TODO Auto-generated catch blocke.printStackTrace();} catch (StreamCorruptedException e) {// TODO Auto-generated catch blocke.printStackTrace();} catch (IOException e) {// TODO Auto-generated catch blocke.printStackTrace();} catch (ClassNotFoundException e) {// TODO Auto-generated catch blocke.printStackTrace();}} /** * 高效读取指定编码格式的文件 * @param path * @return */@SuppressWarnings('resource')public static String FileInput3(String path) {StringBuffer buffer = new StringBuffer();try {BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(new FileInputStream(path), 'UTF-8'));String data = null;while ((data = bufferedReader.readLine()) != null) {buffer.append(data+'rn');} bufferedReader.close();} catch (UnsupportedEncodingException e) {// TODO Auto-generated catch blocke.printStackTrace();} catch (FileNotFoundException e) {// TODO Auto-generated catch blocke.printStackTrace();} catch (IOException e) {// TODO Auto-generated catch blocke.printStackTrace();}return buffer.toString();}}
补充知识:java读取txt文件为List
文件在桌面放着名字为hello.txt,先看一下要读取的内容
这是为了方便展示demo随便写的,格式是一行一个英文单词,一共五个。
读取代码,这个代码也是网上找的,忘了哪个博客了。
import java.io.*;import java.util.ArrayList;import java.util.List; /** * @author : * @date : 2018/8/30 * @description: */public class ReaderFileLine { /** * @author: * @date:2018/8/30 * @description:从txt文件读取List<String> */ public static List<String> getFileContent(String path) { List<String> strList = new ArrayList<String>(); File file = new File(path); InputStreamReader read = null; BufferedReader reader = null; try { read = new InputStreamReader(new FileInputStream(file),'utf-8'); reader = new BufferedReader(read); String line; while ((line = reader.readLine()) != null) {strList.add(line); } } catch (UnsupportedEncodingException e) { e.printStackTrace(); } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } finally { if (read != null) {try { read.close();} catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace();} } if (reader != null) {try { reader.close();} catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace();} } } return strList; } public static void main(String[] args) { List<String> fileContent = ReaderFileLine.getFileContent('C:UsersLenovoDesktophello.txt'); for (String s : fileContent) { System.out.println(s); } } }
输出:
firstsecondThirdFourthFifth
注意:
1.这里File这个类导入的包是Io的,不是Nio的
2. ReaderFileLine.getFileContent('C:UsersLenovoDesktophello.txt'); 这个路径是绝对路径
3.路径是一个 反斜杠 但是在代码里面反斜杠是转义的意思,所以需要再加一个,如果你是用的IDEA恭喜你,它会自动给你加上
以上这篇java 将字符串、list 写入到文件,并读取内容的案例就是小编分享给大家的全部内容了,希望能给大家一个参考,也希望大家多多支持好吧啦网。
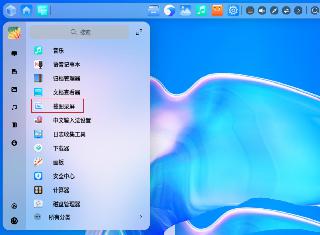