如何用 Python 子进程关闭 Excel 自动化中的弹窗
利用Python进行Excel自动化操作的过程中,尤其是涉及VBA时,可能遇到消息框/弹窗(MsgBox)。此时需要人为响应,否则代码卡死直至超时 [^1] [^2]。根本的解决方法是VBA代码中不要出现类似弹窗,但有时我们无权修改被操作的Excel文件,例如这是我们进行自动化测试的对象。所以本文记录从代码角度解决此类问题的方法。
假想场景使用xlwings(或者其他自动化库)打开Excel文件test.xlsm,读取Sheet1!A1单元格内容。很简单的一个操作:
import xlwings as xwwb = xw.Book(’test.xlsm’)msg = wb.sheets(’Sheet1’).range(’A1’).valueprint(msg)wb.close()
然而不幸的是,打开工作簿时进行了热情的欢迎仪式:
Private Sub Workbook_Open() MsgBox 'Welcome' MsgBox 'to open' MsgBox 'this file.'End Sub
第一个弹窗Welcome就卡住了Excel,Python代码相应卡死在第一行。
主程序中不可能直接处理或者绕过此类问题,也不能奢望有人随时蹲守点击下一步——那就开启一个子线程来护航吧。因此,解决方案是利用子线程监听并随时关闭弹窗,直到主程序圆满结束。解决这个问题,需要以下两个知识点(基础知识请课外学习):
Python多线程(本文采用threading.Thread) Python界面自动化库(本文涉及pywinauto和pywin32) pywinauto方案pywinauto顾名思义是Windows界面自动化库,模拟鼠标和键盘操作窗体和控件 [^3]。不同于先获取句柄再获取属性的传统方式,pywinauto的API更加友好和pythonic。例如,两行代码搞定窗口捕捉和点击:
from pywinauto.application import Applicationwin = Application(backend='win32').connect(title=’Microsoft Excel’)win.Dialog.Button.click()
本文采用自定义线程类的方式,启动线程后自动执行run()函数来完成上述操作。具体代码如下,注意构造函数中的两个参数:
title 需要捕捉的弹窗的标题,例如Excel默认弹窗的标题为Microsoft Excel interval 监听的频率,即每隔多少秒检查一次# listener.pyimport timefrom threading import Thread, Eventfrom pywinauto.application import Applicationclass MsgBoxListener(Thread): def __init__(self, title:str, interval:int):Thread.__init__(self)self._title = title self._interval = interval self._stop_event = Event() def stop(self): self._stop_event.set() @property def is_running(self): return not self._stop_event.is_set() def run(self):while self.is_running: try:time.sleep(self._interval)self._close_msgbox() except Exception as e:print(e, flush=True) def _close_msgbox(self):’’’Close the default Excel MsgBox with title 'Microsoft Excel'.’’’win = Application(backend='win32').connect(title=self._title)win.Dialog.Button.click()if __name__==’__main__’: t = MsgBoxListener(’Microsoft Excel’, 3) t.start() time.sleep(10) t.stop()
于是,整个过程分为三步:
启动子线程监听弹窗 主线程中打开Excel开始自动化操作 关闭子线程import xlwings as xwfrom listener import MsgBoxListener# start listen threadlistener = MsgBoxListener(’Microsoft Excel’, 3)listener.start()# main process as beforewb = xw.Book(’test.xlsm’)msg = wb.sheets(’Sheet1’).range(’A1’).valueprint(msg)wb.close()# stop listener threadlistener.stop()
到此问题基本解决,本地运行效果完全达到预期。但我的真实需求是以系统服务方式在服务器上进行Excel文件自动化测试,后续发现,当以系统服务方式运行时,pywinauto竟然捕捉不到弹窗!这或许是pywinauto一个潜在的问题 [^4]。
win32gui方案那就只好转向相对底层的win32gui,所幸完美解决了上述问题。win32gui是pywin32库的一部分,所以实际安装命令是:
pip install pywin32
整个方案和前文描述完全一致,只是替换MsgBoxListener类中关闭弹窗的方法:
import win32gui, win32condef _close_msgbox(self): # find the top window by title hwnd = win32gui.FindWindow(None, self._title) if not hwnd: return # find child button h_btn = win32gui.FindWindowEx(hwnd, None,’Button’, None) if not h_btn: return # show text text = win32gui.GetWindowText(h_btn) print(text) # click button win32gui.PostMessage(h_btn, win32con.WM_LBUTTONDOWN, None, None) time.sleep(0.2) win32gui.PostMessage(h_btn, win32con.WM_LBUTTONUP, None, None) time.sleep(0.2)更一般的方案
更一般地,当同时存在默认标题和自定义标题的弹窗时,就不便于采用标题方式进行捕捉了。例如
MsgBox 'Message with default title.', vbInformation, MsgBox 'Message with title My App 1', vbInformation, 'My App 1'MsgBox 'Message with title My App 2', vbInformation, 'My App 2'
那就扩大搜索范围,依次点击所有包含确定性描述的按钮(例如OK,Yes,Confirm)来关闭弹窗。同理替换MsgBoxListener类的_close_msgbox()方法(同时构造函数中不再需要title参数):
def _close_msgbox(self): ’’’Click any button ('OK', 'Yes' or 'Confirm') to close message box.’’’ # get handles of all top windows h_windows = [] win32gui.EnumWindows(lambda hWnd, param: param.append(hWnd), h_windows) # check each windowfor h_window in h_windows: # get child button with text OK, Yes or Confirm of given windowh_btn = win32gui.FindWindowEx(h_window, None,’Button’, None)if not h_btn: continue# check button texttext = win32gui.GetWindowText(h_btn)if not text.lower() in (’ok’, ’yes’, ’confirm’): continue# click buttonwin32gui.PostMessage(h_btn, win32con.WM_LBUTTONDOWN, None, None)time.sleep(0.2)win32gui.PostMessage(h_btn, win32con.WM_LBUTTONUP, None, None)time.sleep(0.2)
最后,实例演示结束全文,以后再也不用担心意外弹窗了。
以上就是如何用 Python 子进程关闭 Excel 自动化中的弹窗的详细内容,更多关于Python 子进程关闭 Excel 弹窗的资料请关注好吧啦网其它相关文章!
相关文章:
1. IDEA 2020.1 版自动导入MAVEN依赖的方法(新版MAVEN无法自动导入/更新POM依赖、MAVEN设置自动更新、自动更新快捷键)2. Java多线程通信wait()和notify()代码实例3. 开发Java的又一利器-Visual Cafe4. IDEA 程序包不存在,找不到符号但是明明存在对应的jar包(问题分析及解决方案)5. SublimeText3配置PHP函数追踪定位插件6. 解决vue watch数据的方法被调用了两次的问题7. IDEA标签tabs多行显示的设置8. 详解idea中web.xml默认版本问题解决9. javascript将扁平的数据转为树形结构的高效率算法10. Java实现Windows计算器界面
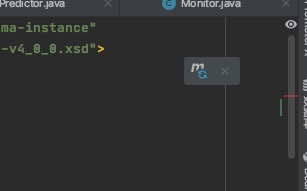